Goals and experience
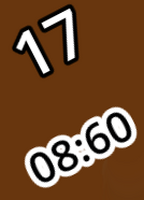
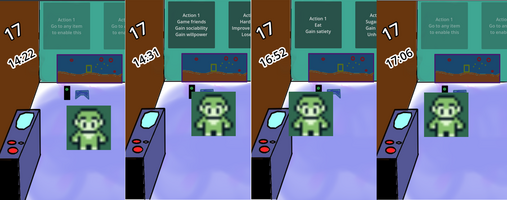
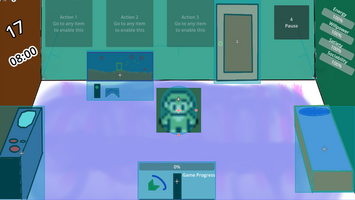
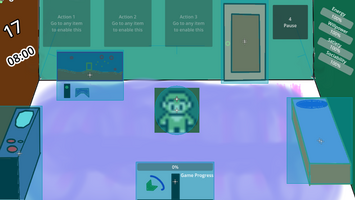
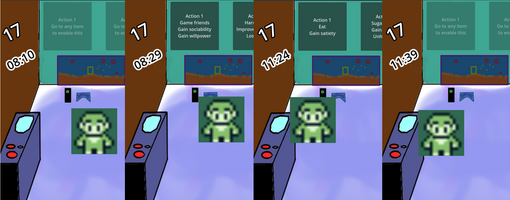
One can set goals for themselves, and that paint the activity they do on a certain way, meaning that different things might have more importance.
For example let's take this game and some of the decisions that were made.
One thing that I wanted to show was the time passing by, whether you did something or not, and of course the passage of time. So one of the first things I implemented was the clock.
If I was to make the game to get more experience on the engine I would have searched for a datetime class or similar, but my goal was another: Finish the game no matter what.
Here I could make use of my experience and in less time than what I would have spent searching for the right data I could implement a good enough alternative that worked for me. What would have been the constraints? Start the 17th at 8:00, end the 31st, increment the time correctly whether we increment it a minute or whatever time we want. With all this I set up to write some code, with the main part being:
@export var hour = 8 @export var minutes = 0 @export var date = 17 func format_time(): if minutes>60 : var inchor = minutes/60 minutes %= 60 hour+=inchor if hour>24 : hour %= 24 date+=1 if date>31 : print("game over")
Mostly I called this function after incrementing time any number of minutes. If you are experienced you might see something that I saw the first time I tried the game with the clock on.
Yeah, that's right, off by one errors. Fortunately I was wise enough to try the edge cases first thing, and solved it immediately.
func format_time(): if minutes>60 : var inchor = minutes/60 minutes %= 60 hour+=inchor if hour>24 : hour %= 24 date+=1 if date>31 : print("game over")
The second thing that you might notice is that the end game condition doesn't seem valid. Don't worry, it is, this is not an end game condition, just a debug message gone wrong, and I'm deleting it right now.
Writing the code was done in less than five minutes, one minute of gameplay to find the bug, 5 seconds to solve it, another minute to test it works well. Less than 10 minutes for a functionality that it could have taken me about that time to find the best way to express time and how to increment it. At least, because as far as I know the main datetime functions in gdScript are about current time, not fantasy time.
How did I do it well? Because I had failed tens of times before. An experienced person is someone who has failed more times than a novice has tried.
Try, fail, learn, try again. That's the way to grow.
Let's examine another problem I found. Each object with which you can interact has a CollisionShape2D that mark their interaction limits, and the character has another CollisionShape2D to mark its position. I used a lame, but fast, way to deduce in which zone the character was. On the Area2D of each object when the character came in (body_entered) I changed _zona to the name of the zone, and changed _zona to an empty string when the character left (body_exited). What was the problem? That some objects where too close to one another, and the character entered one without exiting the previous one, and exiting the previous one they lost the current one. Let's see it in play.
What did I do? On body_exited I only set _zona to blank if it was equal to the object being exited. And it worked. But only the other day I thought that it only works for the usual case, in where the character is moving from object A to B, not when it moves from A to B and back to A without exiting A.
I thought how could I solve that, and started thinking about implementing a list in _zona instead of a string, and other complicated things, until I remembered that frontend exists for a reason. An experienced game developer would have found the better solution much sooner. Just move the zones away so it's not possible for this to happen.
Some displacement of the objects, a bit of resize for the bed area and the character area, and problem solved for good in any case.
To summarize whatever goal you have will affect how you do things, experience is what you get when you learn, and failing is a valid strategy for learning.
Get SoloGameDev
SoloGameDev
Solo Game Developer Jamming
Status | Released |
Author | JaumeGreen |
Genre | Simulation |
Tags | Godot, Short, Singleplayer |
Languages | English |
More posts
- Differing opinionsAug 15, 2024
- Technical Debt is a harmful termAug 06, 2024
- Dreams versus realityAug 02, 2024
- Language, engine, and dubious practicesAug 01, 2024
- Why SoloGameDev?Jul 31, 2024
Leave a comment
Log in with itch.io to leave a comment.